How To use 7 Segment Display explained
In this lesson, we will program the Arduino to achieve the controlling of a segment display.
How To use 7 Segment Display explained
LED segment displays are common for displaying numerical information. It’s widely applied on displays of electromagnetic oven, full automatic washing machine, water temperature display, electronic clock etc.
Table of Contents
Hardware Required
- 1 * Arduino UNO
- 1 * USB Cable
- 1 * 220 Ω Resistor
- 1 * 7-segment Display
- 1 * Breadboard
- Several jumper wires
Circuit Connection
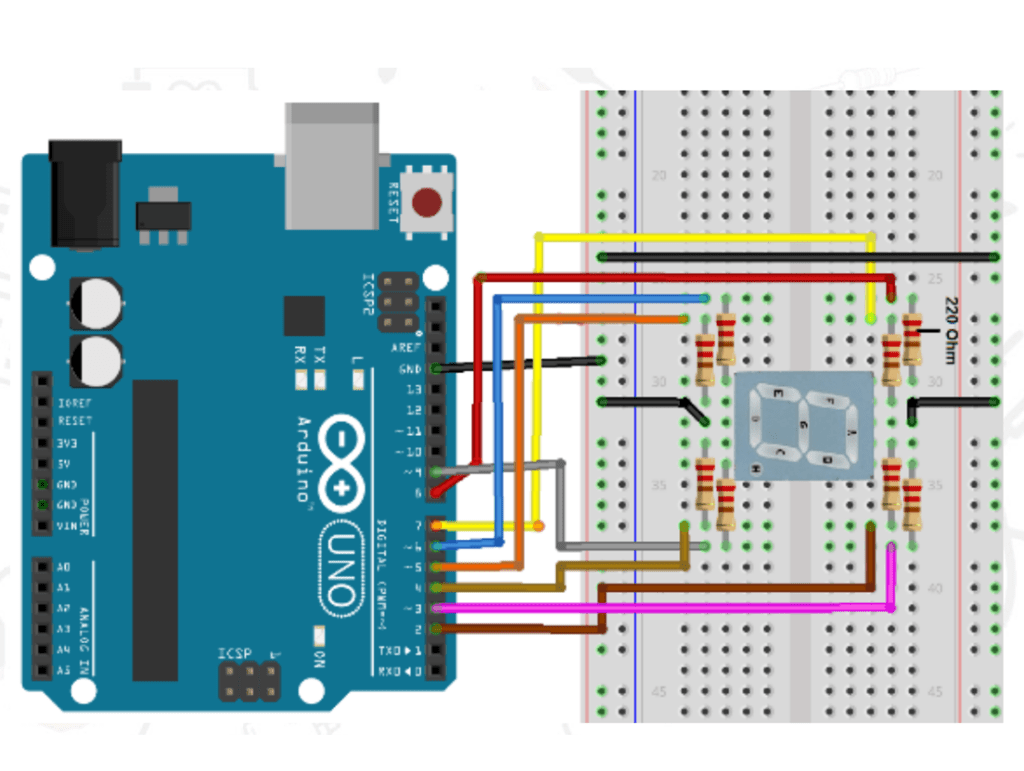
First, attach the 1 Digit (7 Segment) Display on the Breadboard.
- Connect GND of Arduino UNO with Breadboard for making further GND connections.
- Connect both the GND of 1 Digit (7 Segment) Display with the GND rail of the Breadboard.
- Connect pins of 1 Digit (7 Segment) Display with Arduino UNO via 220 Ω resistor as described in the table below:
Digit (7 Segment) | Arduino UNO |
LED 1 (A) | Digital Pin 2 |
LED 2 (B) | Digital Pin 3 |
LED 3 (C) | Digital Pin 4 |
LED 4 (D) | Digital Pin 5 |
LED 5 (E) | Digital Pin 6 |
LED 6 (F) | Digital Pin 7 |
LED 7 (G) | Digital Pin 8 |
LED 8 (H) | Digital Pin 9 |
Working and Output
Welcome to the Arduino Based Project, which consists of Eight Segment Display. The LED segment display is a semiconductor light-emitting device. Its basic unit is a light-emitting diode (LED). LED segment display
can be divided into 7-segment display and 8-segment display according to the number of segments. 8-segment display has one more LED unit (for decimal point display) than 7-segment one. In this experiment, we use 8-segment display.
- According to the wiring method of LED units, LED segment displays can be divided into display with common anode and display with common cathode.
- Common anode display refers to the one that combine all the anodes of LED units into one common anode (COM).
- For the common anode display, connect the common anode (COM) to +5V.
- When cathode level of a certain segment is low, the segment is on; when cathode level of a certain segment is high, the segment is off.
- For the common cathode display, connect the common cathode (COM) to GND.
- When the anode level of a certain segment is high, the segment is on; when the anode level of a certain segment is low, the segment is off. It can have Common cathode 7-segment display and Common anode 7-segment display
Each segment of the display consists of an LED. So when you use it, you also need to use a current-limiting resistor. Otherwise, LED will be burnt out. In this project, we use a common cathode display. As we mentioned above, for common cathode display, connect the common cathode (COM) to GND.
When the anode level of a certain segment is high, the segment is on; when the anode level of a certain segment is low, the segment is off. There are seven segments for numerical display, one for decimal
point display. Corresponding segments will be turned on when displaying certain numbers. For example, when displaying number 1, b and c segments will be turned on. We compile a subprogram for each
number, and compile the main program to display one number every 2 seconds, cycling display number 0 ~ 9. The displaying time for each number is subject to delay time, the longer delay time, the longer displaying time. LED segment display displays number 0 to 9.
7 Segment Display Arduino Code
// uno onboard LED @ pin 13:
// name the pins. DP is not used in this project.
int A = 5;
int B = 6;
int C = 7;
int D = 2;
int E = 3;
int F = 4;
int G = 9;
int H = 8;
// make the functions.
// see .txt page for HIGH/LOW schedules.
void zero()
{
digitalWrite(5, HIGH);
digitalWrite(6, HIGH);
digitalWrite(7, HIGH);
digitalWrite(2, HIGH);
digitalWrite(3, HIGH);
digitalWrite(4, HIGH);
digitalWrite(9, LOW);
digitalWrite(8, LOW);
delay(1000);
}
void nine()
{
digitalWrite(A, HIGH);
digitalWrite(B, HIGH);
digitalWrite(C, HIGH);
digitalWrite(D, HIGH);
digitalWrite(E, LOW);
digitalWrite(F, HIGH);
digitalWrite(G, HIGH);
digitalWrite(H, HIGH);
delay(1000);
}
void eight()
{
digitalWrite(A, HIGH);
digitalWrite(B, HIGH);
digitalWrite(C, HIGH);
digitalWrite(D, HIGH);
digitalWrite(E, HIGH);
digitalWrite(F, HIGH);
digitalWrite(G, HIGH);
digitalWrite(H, HIGH);
delay(1000);
}
void seven()
{
digitalWrite(A, HIGH);
digitalWrite(B, HIGH);
digitalWrite(C, HIGH);
digitalWrite(D, LOW);
digitalWrite(E, LOW);
digitalWrite(F, LOW);
digitalWrite(G, LOW);
digitalWrite(H, HIGH);
delay(1000);
}
void six()
{
digitalWrite(A, HIGH);
digitalWrite(B, LOW);
digitalWrite(C, HIGH);
digitalWrite(D, HIGH);
digitalWrite(E, HIGH);
digitalWrite(F, HIGH);
digitalWrite(G, HIGH);
digitalWrite(H, HIGH);
delay(1000);
}
void five()
{
digitalWrite(A, HIGH);
digitalWrite(B, LOW);
digitalWrite(C, HIGH);
digitalWrite(D, HIGH);
digitalWrite(E, LOW);
digitalWrite(F, HIGH);
digitalWrite(G, HIGH);
digitalWrite(H, HIGH);
delay(1000);
}
void four()
{
digitalWrite(A, LOW);
digitalWrite(B, HIGH);
digitalWrite(C, HIGH);
digitalWrite(D, LOW);
digitalWrite(E, LOW);
digitalWrite(F, HIGH);
digitalWrite(G, HIGH);
digitalWrite(H, HIGH);
delay(1000);
}
void three()
{
digitalWrite(A, HIGH);
digitalWrite(B, HIGH);
digitalWrite(C, HIGH);
digitalWrite(D, HIGH);
digitalWrite(E, LOW);
digitalWrite(F, LOW);
digitalWrite(G, HIGH);
digitalWrite(H, HIGH);
delay(1000);
}
void two()
{
digitalWrite(A, HIGH);
digitalWrite(B, HIGH);
digitalWrite(C, LOW);
digitalWrite(D, HIGH);
digitalWrite(E, HIGH);
digitalWrite(F, LOW);
digitalWrite(G, HIGH);
digitalWrite(H, HIGH);
delay(1000);
}
void one()
{
digitalWrite(A, LOW);
digitalWrite(B, HIGH);
digitalWrite(C, HIGH);
digitalWrite(D, LOW);
digitalWrite(E, LOW);
digitalWrite(F, LOW);
digitalWrite(G, LOW);
digitalWrite(H, HIGH);
delay(1000);
}
void dot()
{
digitalWrite(5, LOW);
digitalWrite(6, LOW);
digitalWrite(7, LOW);
digitalWrite(2, LOW);
digitalWrite(3,LOW);
digitalWrite(4, LOW);
digitalWrite(9, LOW );
digitalWrite(8, HIGH);
delay(1000);
}
/* The setup() function is called when a sketch starts. It is used to initialize variables, pin modes, start using libraries, etc. This function will only run once, after each power up or reset of the Arduino board. */
void setup()
{
pinMode(5, OUTPUT);
pinMode(6, OUTPUT);
pinMode(7, OUTPUT);
pinMode(2, OUTPUT);
pinMode(3, OUTPUT);
pinMode(4, OUTPUT);
pinMode(9, OUTPUT);
pinMode(8, OUTPUT);
}
/* This Particular Function is used for Repeated Execution of the Circuit until Specified. */
void loop()
{
zero();
nine();
eight();
seven();
six();
five();
four();
three();
two();
one();
dot ();
}
7 Segment Display connections
Common Anode: All the Negative terminals (cathode) of all the 8 LEDs are connected together. All the positive terminals are left alone.
Common Cathode: All the positive terminals (anode) of all the 8 LEDs are connected together. All the negative thermals are left alone.
Common Anode Seven Segment Display
In common anode type, all the anodes of 8 LEDs are connected to the common terminal and cathodes are left free. Thus, in order to glow the LED, these cathodes have to be connected to the logic ‘0’ and
anode to the logic ‘1’. Below, the truth table gives the information required for driving the common anode seven segments.
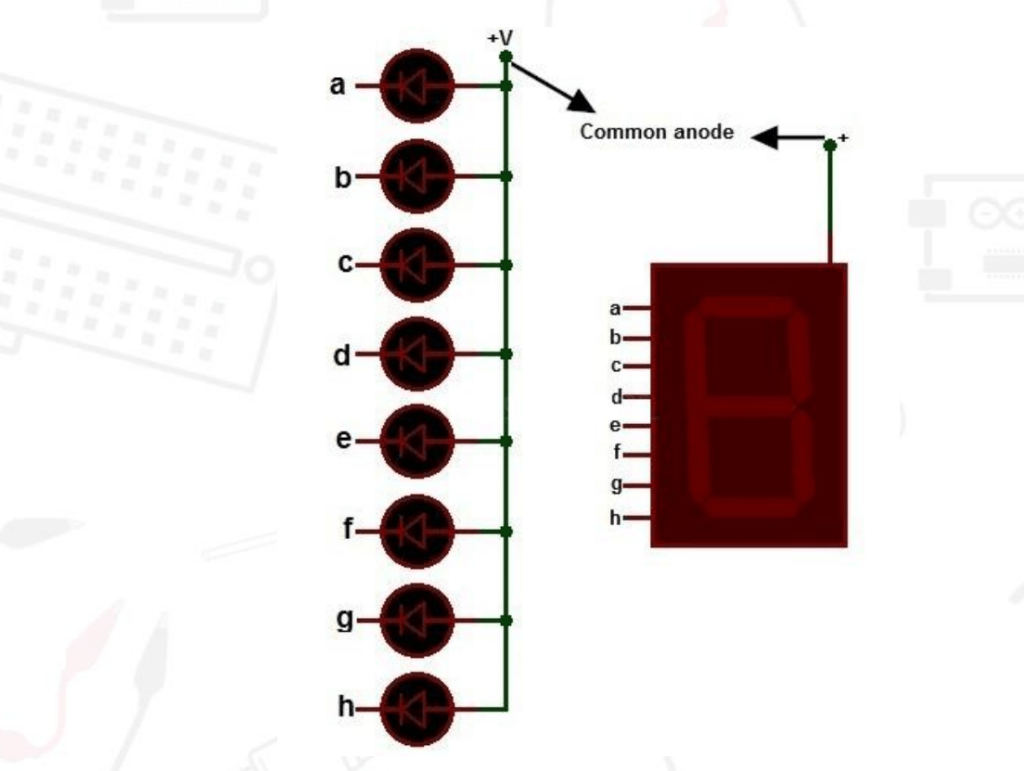
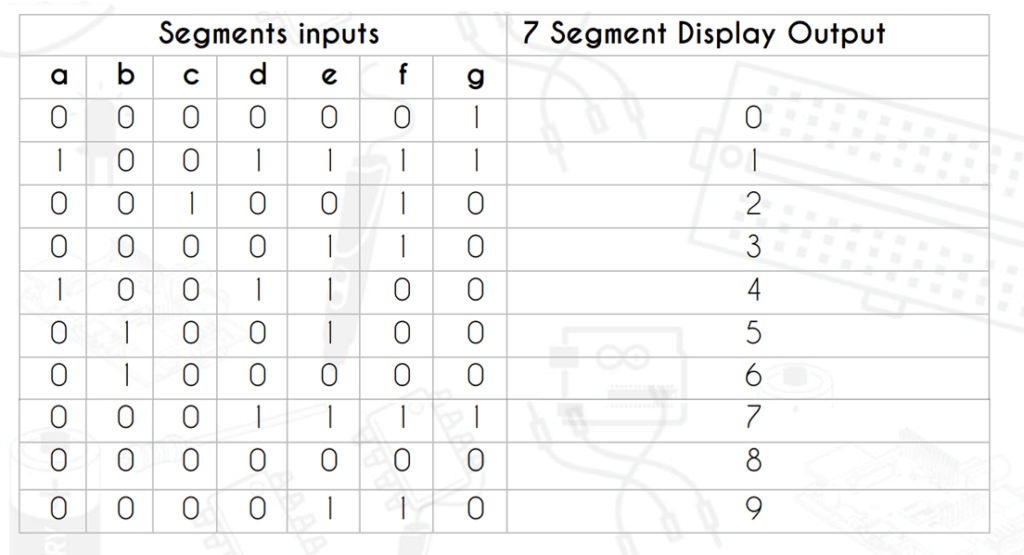
Common Cathode Seven Segment Display
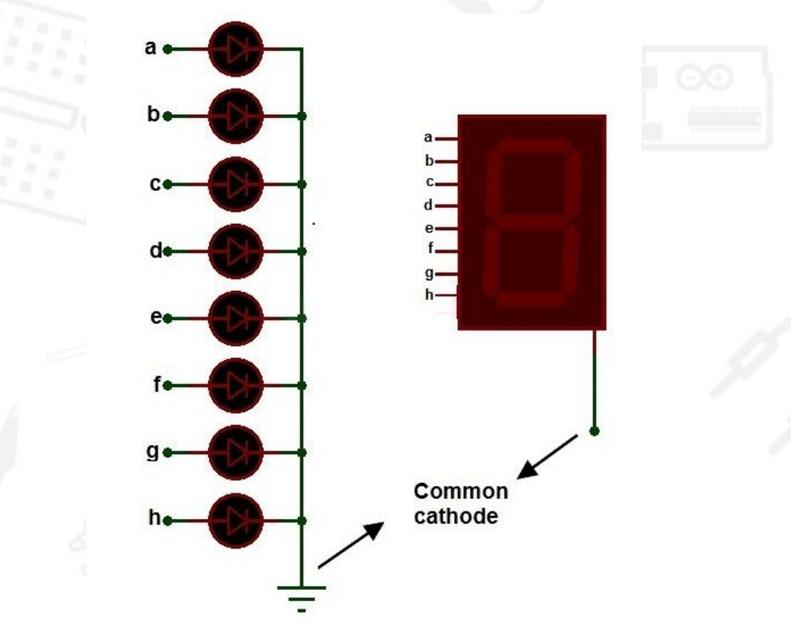
As the name indicates, cathode is the common pin for this type of seven segments and the remaining 8 pins are left free. Here, logic low is applied to the common pin and logic high to the remaining pins.
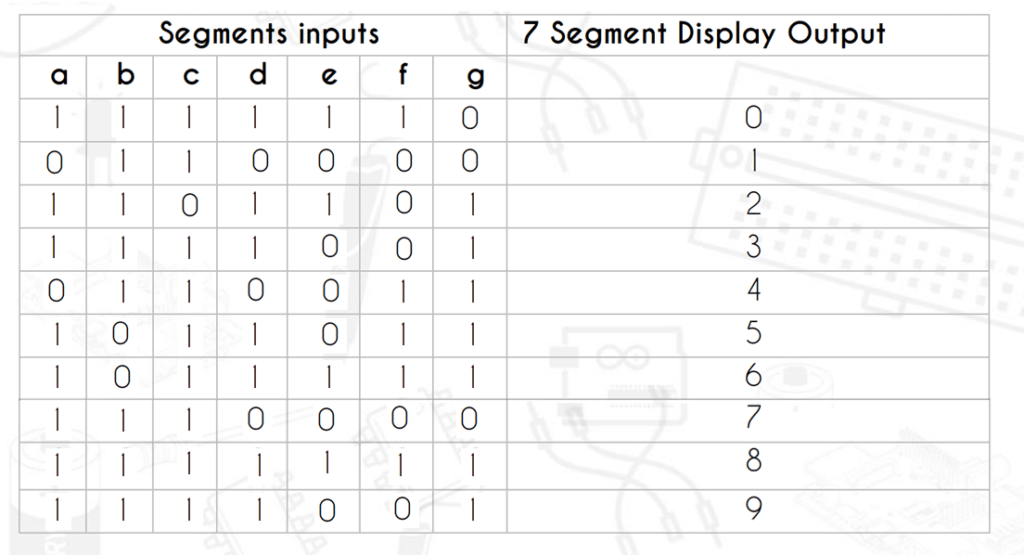
The above truth table shows the data to be applied to the seven segments to display the digits. In order to display digit ‘0’ on seven segments, segments a, b, c, d, e and f are applied with logic high and segment g is applied with logic low.
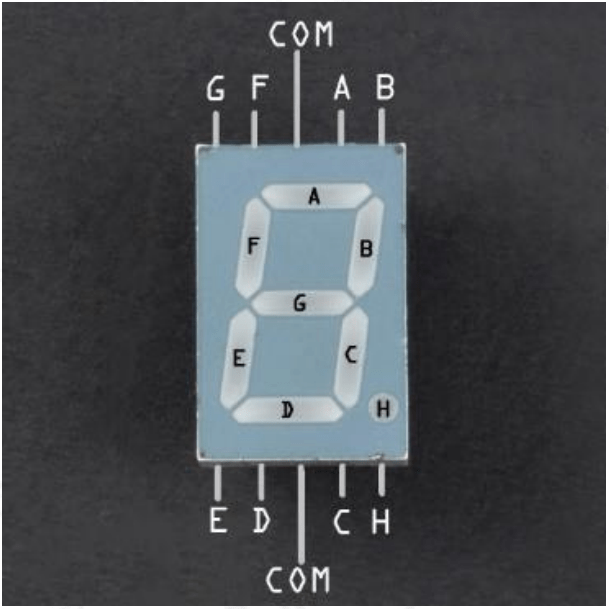